Overview
Appsmith Workflows provide you with an intuitive interface to connect with external services, automate tasks, and orchestrate process within your applications. They integrate code-based and node-based systems. By simplifying complex tasks with JavaScript logic, Appsmith Workflows are ideal for efficient and advanced workflow automation.
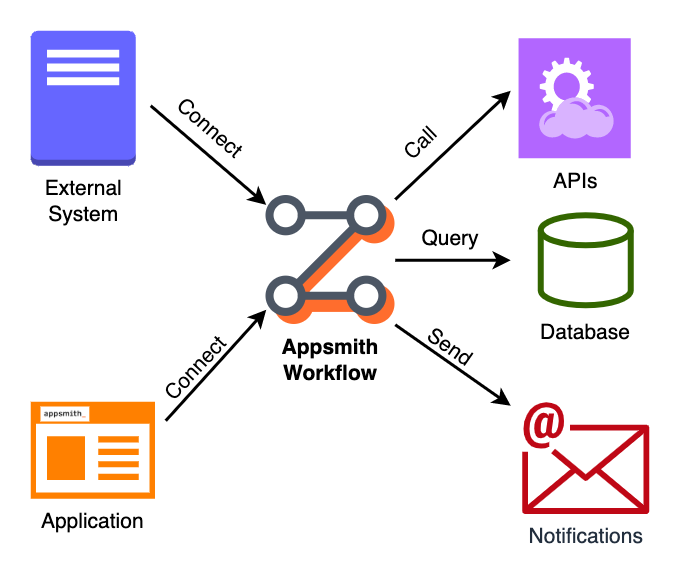
Appsmith Workflows are versatile and designed to handle a wide range of scenarios:
-
Automate business-critical processes: Streamline operations by automating tasks such as: syncing leads from CRM to marketing tools, or managing inventory with stock alerts and supplier orders.
-
Enable Human-in-the-Loop interactions: Combine user-friendly interfaces with server-side automation to design workflows that require human decisions for: Data validation, Approvals, Feedback, and more. (Coming Soon)
-
Explore trigger types: Appsmith offers different trigger types including Webhooks, Integrated Datasource for Appsmith Apps, and Scheduled Cron Jobs.
Getting started with workflows
Learning the fundamentals of Appsmith Workflows is crucial for maximizing productivity and building robust applications. Begin exploring Appsmith Workflows with the comprehensive getting started tutorial.